|
|
Line 1: |
Line 1: |
| + | == Introduction == |
| + | Authorization Code Flow<br> |
| + | [[File:CMUOAuth-authozizationcode flow.jpg|link=]]<br> |
| + | from [https://oauth.cmu.ac.th CMU OAuth] |
| == Methods == | | == Methods == |
| === Constructor === | | === Constructor === |
Revision as of 08:26, 25 September 2017
Introduction
Authorization Code Flow
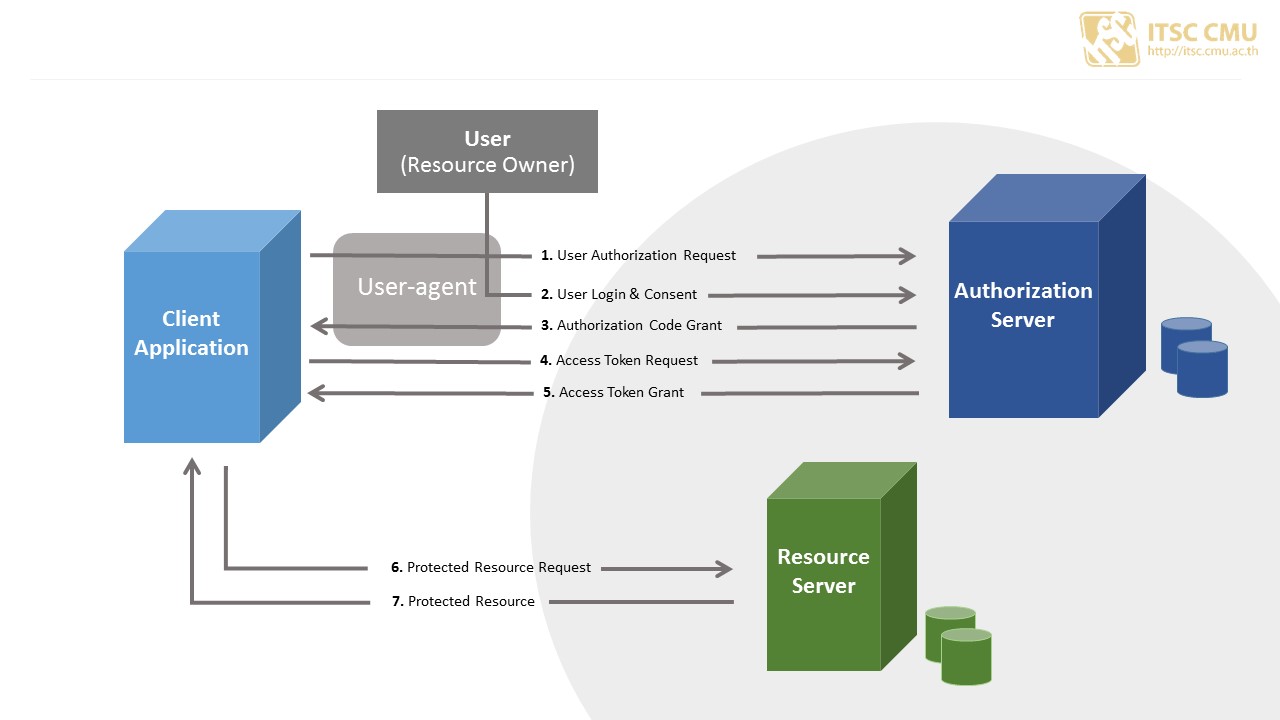
from CMU OAuth
Methods
Constructor
Set Client ID, Client Secret, Redirect URI
Description
__construct([string $appId, string $clientSecret, string $redirectURI])
|
Parameters
name |
description
|
appId
|
cmu oauth Client ID
|
clientSecret
|
cmu oauth Client Secret
|
redirectURI
|
cmu oauth Redirect URI
|
Return Values
no return value
|
setAppId
set Client ID
Description
setAppId(string $appid)
|
Parameters
name |
description
|
appid
|
cmu oauth Client ID
|
Return Values
no return value
|
setAppSecret
Set Client Secret
Description
setAppSecret(string $appSecret)
|
Parameters
name |
description
|
appSecret
|
cmu oauth Client Secret
|
Return Values
no return value
|
setCallbackUri
Set Redirect URI
Description
setCallbackUri(string $uri)
|
Parameters
name |
description
|
uri
|
Application Callback / Redirect URI
|
Return Values
no return value
|
initOauth
Initial redirect to CMU Oauth for authorization.
Return Values
no return value
|
getAccessToken
Get user's authorized access token.
Description
object getAccessToken(string $code)
|
Parameters
name |
description
|
code
|
code that parse by CMU Oauth to redirect URI.
|
Return Values
object
|
{
"access_token": "66822448858031556636",
"expires_in": 3600,
"refresh_token": "23178027621214615262"
}
|
getUserInfo
Get user's information by user's authorized access token.
Description
object getUserInfo(string $accessToken)
|
Parameters
name |
description
|
accessToken
|
user's authorized access token
|
Return Values
object
|
{
"status": true,
"data": {
"timestamp": "2017-03-31T17:30:55.7933253+07:00",
"itaccount_name": "jon_s",
"citizen_id": "1111111111111",
"student_id": "520510999",
"prefix": {
"en_US": "Mr.",
"th_TH": "นาย"
},
"first_name": {
"en_US": "JON",
"th_TH": "จอน"
},
"last_name": {
"en_US": "SNOW",
"th_TH": "สโนว์"
},
"organization": {
"code": "05",
"name": {
"en_US": "Faculty of Science",
"th_TH": "คณะวิทยาศาสตร์"
}
},
"itaccount_type": {
"id": "AlumAcc",
"en_US": "Alumni Account",
"th_TH": "นักศึกษาเก่า"
}
}
}
|
Examples
callback.php
<?php
// provide your application id,secret and redirect uri
$appId = 'your cmu ouath client ID';
$appSecret = 'your cmu oauth client secret';
$callbackUri = 'your cmu oauth Redirect URI';
require('cmu.oauth.class.php');
// new CMU Oauth Instance.
$cmuOauth = new cmuOauth();
// set your application id,secret and redirect uri
$cmuOauth->setAppId($appId);
$cmuOauth->setAppSecret($appSecret);
$cmuOauth->setCallbackUri($callbackUri);
if(isset($_GET['code'])){
// code parse from CMU Oauth to your redirect uri.
$code = $_GET['code'];
// get access token from code.
$accessToken = $cmuOauth->getAccessToken($code);
// get user information from access token.
$userInfo = $cmuOauth->getUserInfo($accessToken->access_token);
// do login process
// create session if status == true, refer to return values of cmuOauth::getUserInfo
// else destroy session
if($userInfo->status===true){
session_start();
$sid = session_id();
$_SESSION["user_$sid"]=$userInfo->data->itaccount_name."@cmu.ac.th";
header("location: https://example.com/main.html");
exit();
}else {
session_start();
unset($_SESSION["user_$sid"]);
session_destroy();
header("location: https://example.com/403.html");
exit();
}
}else{
// initial redirect to CMU Oauth login page.
$cmuOauth->initOauth();
}
?>
Download
cmu.oauth.class.php.zip
Reference
https://oauth.cmu.ac.th
https://tools.ietf.org/html/rfc6749